Today, another good point emerged in the comments section of our Kickstarter campaign – a question arises as to whether it is possible to utilize the Dice Device as a tool for teaching the fundamentals of programming.
The answer is yes and I’m actually applying it on my kid ;-). But let me elaborate a bit more. Part of Dice Device project is an open source development framework where adding own application is as simple as adding one file to the project. In the file there is one class represeting the application (the language is C++) and there a programmer can define what to do during application start, during execution and during exit. And that’s basically it. Let me present here the complete code of a simple Hello World application:
#include <memory>
#include "Arduino.h"
#include "lvgl.h"
#include "..\..\menu\ddApp.h"
#include "stdlib.h"
//! Hello World Application
class AppHelloWorld : public DDApp
{
//! Hello World text to be displayed in the center of the screen
lv_obj_t* _text;
public:
//! Constructor
AppHelloWorld(lv_obj_t* background) : DDApp(background)
{
_text = lv_label_create(lv_scr_act());
lv_obj_align(_text, LV_ALIGN_CENTER, 0, 0);
lv_label_set_text(_text, "Hello World!");
}
//! Destructor
~AppHelloWorld()
{
lv_obj_del(_text);
}
//! Simulation step, invoked every rendering frame
void Tick(int tp, bool pressed, bool depressed, int x, int y)
{
if (pressed)
lv_label_set_text(_text, "Screen Was Touched");
}
};
This code starts the application with Hello World! text in the center of the screen and after touching the screen it changes to Screen Was Touched text. This is what user sees:
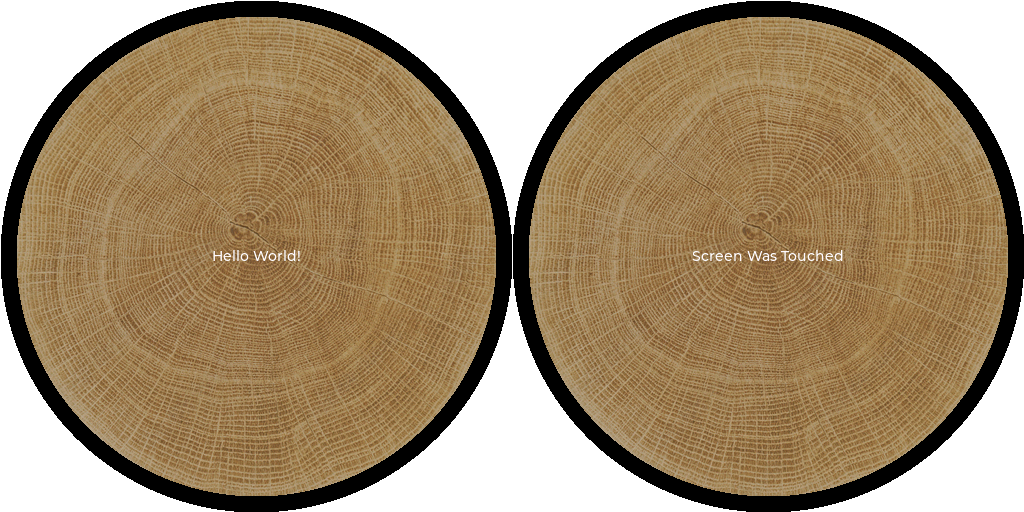
I’m sure that somebody could admit if C++ is a good starting language for teaching basics of programming. My personal answer is yes. What is yours? 😉